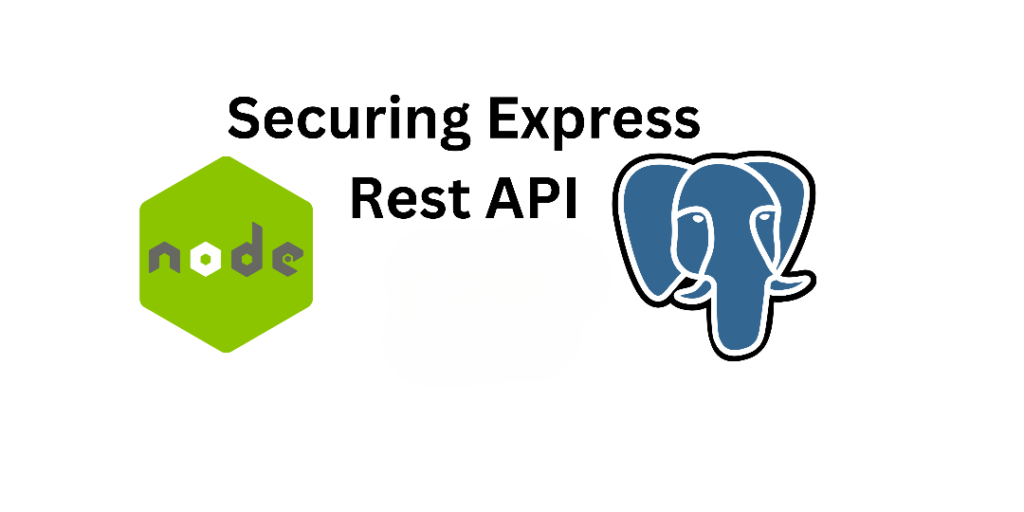
Introduction
The MERN stack is a popular JavaScript-based technology stack used for building full-stack web applications. It consists of MongoDB (database), Express.js (backend framework), React.js (frontend library), and Node.js (runtime environment). Together, these technologies enable developers to build dynamic, scalable, and high-performance web apps using a single language—JavaScript. Refer to the MERN Stack Course Online to learn more. At the heart of this stack, the Express API plays a crucial role in managing server-side operations, handling requests, and connecting the frontend to the database.
What Is An Express API?
An Express API is a backend service built using Express.js, a fast and minimalist Node.js framework. In the MERN stack (MongoDB, Express.js, React, Node.js), the Express API acts as the middleware layer that handles HTTP requests and connects the React frontend with the MongoDB database. It defines endpoints (routes) for operations like creating, reading, updating, or deleting data (CRUD).
This API processes incoming requests from the React app, performs business logic, interacts with MongoDB via Mongoose, and sends responses back. It’s essential for managing data flow and implementing server-side logic in a MERN-based full-stack application.
How To Secure An Express API?
Securing an Express API is a critical step in ensuring your application is safe from unauthorized access, data breaches, and malicious attacks. Express.js, being a minimal and flexible Node.js framework, provides various ways to secure your backend API.
Below is a detailed overview of strategies and techniques to enhance the security of an Express API.
1. Use HTTPS
Always use HTTPS instead of HTTP to encrypt data in transit between clients and the server. This prevents eavesdropping and man-in-the-middle attacks. In production environments, HTTPS can be enforced using reverse proxies like NGINX or directly using the https module in Node.js.
2. Helmet Middleware
Helmet is a widely used middleware that helps secure Express apps by setting various HTTP headers. It includes protection against common vulnerabilities like XSS, clickjacking, and MIME sniffing.
“const helmet = require(‘helmet’);
app.use(helmet());”
This adds headers such as Content-Security-Policy, X-Frame-Options, and more to harden your app.
3. Authentication and Authorization
Use secure methods for authentication, such as:
- JWT (JSON Web Tokens) for stateless authentication.
- OAuth2 for third-party access.
- Session-based authentication for stateful APIs.
Always validate tokens on each request and restrict access to certain endpoints based on user roles. Refer to the Mern Stack Course in Hyderabad for more information on MERN Stack and Express API.
“const jwt = require(‘jsonwebtoken’);
function authenticateToken(req, res, next) {
const token = req.headers[‘authorization’]?.split(‘ ‘)[1];
if (!token) return res.sendStatus(401);
jwt.verify(token, process.env.JWT_SECRET, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
}”
4. Input Validation and Sanitization
Validate all incoming data to prevent SQL injection, NoSQL injection, or other attacks. Use libraries like:
- express-validator
- Joi
- js
Example with express-validator:
“const { body, validationResult } = require(‘express-validator’);
app.post(‘/user’,
body(’email’).isEmail(),
(req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Proceed with safe data
});”
5. Rate Limiting and Throttling
Prevent brute-force attacks by limiting the number of requests from a single IP. Use express-rate-limit:
“const rateLimit = require(‘express-rate-limit’);
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP
});
app.use(limiter);”
6. CORS Configuration
Properly configure Cross-Origin Resource Sharing (CORS) to control which domains can access your API.
“const cors = require(‘cors’);
const corsOptions = {
origin: [‘https://example.com’],
methods: [‘GET’, ‘POST’],
};
app.use(cors(corsOptions));”
7. Avoid Information Leakage
Never expose stack traces or error messages to the client. Customize error handling to log details internally and return generic error messages externally. One can check MERN Stack Training in Noida for the best guidance.
“app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send(‘Something went wrong!’);
});”
8. Secure Data Storage
Always hash sensitive data like passwords using libraries such as bcrypt before storing them in the database.
“const bcrypt = require(‘bcrypt’);
const hashedPassword = await bcrypt.hash(password, 10);”
Conclusion
Securing an Express API involves a layered approach—encrypting traffic, validating inputs, managing access, and configuring security headers. By implementing practices like HTTPS, Helmet, JWT authentication, rate limiting, and input validation, developers can significantly reduce the attack surface of their applications. Staying updated with security best practices and conducting regular audits is crucial for long-term protection.